Get the app here. https://itunesconnect.apple.com/WebObjects/iTunesConnect.woa/wo/5.0.9.7.7.3.1.1.0.5.3.1.1
Monday, August 2, 2010
Learn English on the iPhone
Get the app here. https://itunesconnect.apple.com/WebObjects/iTunesConnect.woa/wo/5.0.9.7.7.3.1.1.0.5.3.1.1
Sunday, July 25, 2010
Short post on navigating between views.
Monday, July 19, 2010
I am on AppStore
Finally, my first app on AppStore. Check it out.
Useful tool to use before deciding to buy that Punggol HDB flat or Weekend car
http://itunes.apple.com/sg/app/easy-loan-calculator/id381932538?mt=8
Sunday, July 18, 2010
Reading a Text File line by line and Tokenizing each line in Objective C
Thursday, July 15, 2010
Ask Octopus Paul
Using Two PickerViews together
Expanding upon the previous tutorial, we will explore how to use two PickerViews together. Such is useful for ‘converter’ like applications, eg. currency converter, units converter.
Below figure shows an example of a bible units converter. that uses two PickerViews.
To have two PickerViews in your app, first drag and drop another PickerView into you xib file .. Declare the Outlet for it. Remember to click them to File’s owner.
The code where you have to edit are the below. Include an if-else condition based on the pickerView that triggered the method. I have two pickerViews, one named pickerView, the other pickerView2. Check which pickerView triggered the method, and set the corresponding label to the value of that particular pickerView.
- (void)pickerView:(UIPickerView *)thePickerView didSelectRow:(NSInteger)row inComponent:(NSInteger)component
{
if([thePickerView isEqual:self.pickerView])
mlabel.text= [arrayNo objectAtIndex:row];
else
mlabel2.text= [arrayNo2 objectAtIndex:row];
}
Have a similar if-else condition to return the no. of rows in each PickerView and also the title for each pickerView cell.
- (NSInteger)pickerView:(UIPickerView *)pickerView numberOfRowsInComponent:(NSInteger)component;
{
if([pickerView isEqual:self.pickerView])
return [arrayNo count];
else
return [arrayNo2 count];
}
- (NSString *)pickerView:(UIPickerView *)pickerView titleForRow:(NSInteger)row forComponent:(NSInteger)component;
{
if([pickerView isEqual:self.pickerView])
return [arrayNo objectAtIndex:row];
else
return [arrayNo2 objectAtIndex:row];
}
So there you go, how to use two picker Views together.
Monday, July 12, 2010
Playing Sound with button clicks - Vuvuzela App
#import <UIKit/UIKit.h>
#include <AudioToolbox/AudioToolbox.h>
@interface SysSoundViewController : UIViewController {
CFURLRef soundFileURLRef;
SystemSoundID soundFileObject;
}
@property (readwrite) CFURLRef soundFileURLRef;
@property (readonly) SystemSoundID soundFileObject;
- (IBAction) playASound;
- (IBAction) vibrate;
@end
Explanation:
We declare a CFURLRef which is a reference to a object representing access to a URL. It is useful as long as the resource you are accessing can be done via a URL. This CSURL object will later hold the URL to our sound files.
Copy the following code next for the ViewController.m file.
#import "SysSoundViewController.h"
@implementation SysSoundViewController
@synthesize soundFileURLRef;
@synthesize soundFileObject;
CFBundleRef mainBundle;
- (void) viewDidLoad {
[super viewDidLoad];
// Get the main bundle for the app
mainBundle = CFBundleGetMainBundle ();
}
// Respond to a tap on the System Sound button
- (IBAction) playASound {
// Get the URL to the sound file to play
soundFileURLRef = CFBundleCopyResourceURL (mainBundle,CFSTR ("a"),CFSTR("wav"),NULL);
// Create a system sound object representing the sound file
AudioServicesCreateSystemSoundID (soundFileURLRef, &soundFileObject);
AudioServicesPlaySystemSound (self.soundFileObject);
}
// Respond to a tap on the Vibrate button
- (IBAction) vibrate {
AudioServicesPlaySystemSound (kSystemSoundID_Vibrate);
}
- (void) dealloc {
[super dealloc];
AudioServicesDisposeSystemSoundID (self.soundFileObject);
CFRelease (soundFileURLRef);
}
@end
Explanation:
The CFBundle allows you to use a folder hierachy called a bundle to organize and locate serveral kinds of resources like images, sounds or executable code. In our case, it represents the folder hierachy we have in the 'Resources' folder. Essentially, it provides us access to our Resources folder.
Sunday, July 11, 2010
Make your own Vuvuzela app
It’s an app that’s been downloaded 5 million times. So what’s so special about it? Other similar examples are the iFart app.
Essentially, both apps are just buttons with an image that plays a sound when clicked. So how hard can it be?
Will go through how to play an audio file soon. And you can be on your way to doing your own vuvuzela app.
Saturday, July 10, 2010
Making the keyboard disappear - Easy Loan Calculator Part 4
Surprisingly, the return/done key does not allow us to close the keyboard.
What we aim to achieve to do here is whenever the user clicks outside of any textbox, the key board will disappear. How do we do that?
In Interface Builder, create a button and stretch it over the entire screen. It should cover the entire screen. Go to Layout -> Send to back. The button should be send to the back.
Hence, whenever a user clicks outside the textbox, she is actually clicking on the background button.
Remember that we have actually declared an Action in the .h file.
-(IBAction) bgTouched:(id) sender;
We have also implemented it in the .m file.
Friday, July 9, 2010
Using Interface Builder to connect Outlets/Actions - Easy Loan Calculator Part 3
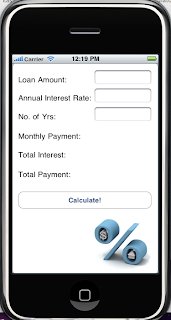